Python has a module (Flask) that allows to run a Python module from the commmand line. The code looks like:
# import main Flask class and request object from flask import Flask, request # create the Flask app app = Flask(__name__) @app.route('/form-example', methods=['GET', 'POST']) def form_example(): if request.args.get('new') == 'NEW': return ''' <form method="POST" action ="http://127.0.0.1:5000/form-example"> <div><label>Language: <input type="text" name="language"></label></div> <div><label>Framework: <input type="text" name="framework"></label></div> <input type="submit" value="Submit"> </form>''' language = request.form.get('language') framework = request.form.get('framework') return ''' <h1>The language value is: {}</h1> <h1>The framework value is: {}</h1>'''.format(language, framework)
This can be run from the command line with: python index.py, which leads to a service that is started.
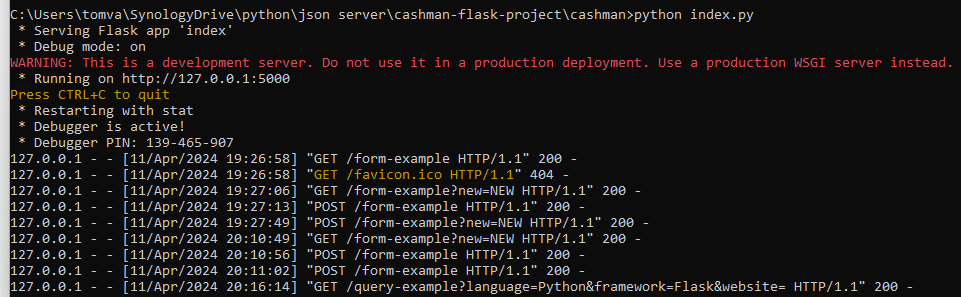
Look at the code. If we provide an URL like “http://127.0.0.1:5000/form-example?new=NEW”, we see that two input fields are provided:
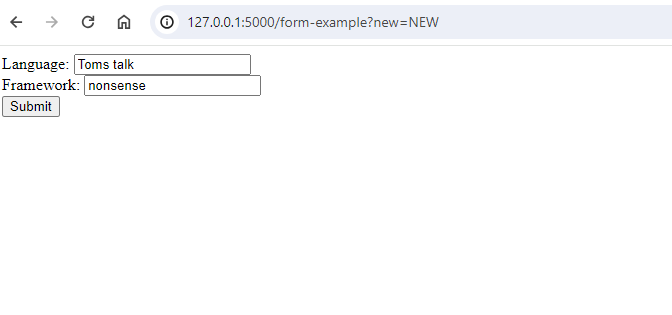
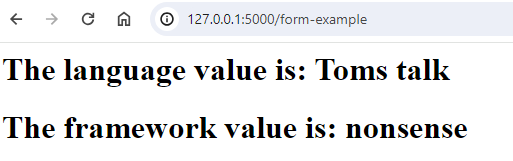