One may use the node.js server as a means to get acquainted with restful services. Let us assume this server is installed and started with
json-server "C:\Users\tomva\SynologyDrive\python\json server\db.json" --port 8000
We can then see the json database within the browser “http://localhost:8000/extra”. We may add some information by:
import requests import json api_url = "http://localhost:8000/extra" todo = {"userId": 1, "title": "Buy nore sugar", "completed": False} headers = {"Content-Type":"application/json"} response = requests.post(api_url, data=json.dumps(todo), headers=headers) print(response.json()) print(response.status_code)
Data is added with ‘id’: ‘8701’. This id is important if one would like to change some data. Look at the put methode that is used below:
import requests import json url = 'http://localhost:8000/extra/8701' data = {"id": 3,"userId": 2, "title": "drink beer", "body": "drinking beer is nice"} headers = {'Content-Type':'application/json; charset=UTF-8'} response = requests.put(url, data=json.dumps(data), headers=headers) print(response.status_code) print(response.text)
The data can be retrieved with the get method:
import requests api_url = "http://localhost:8000/extra/8701" response = requests.get(api_url) response.json() print(response.text) print(response.status_code)
The response.text contains the data:
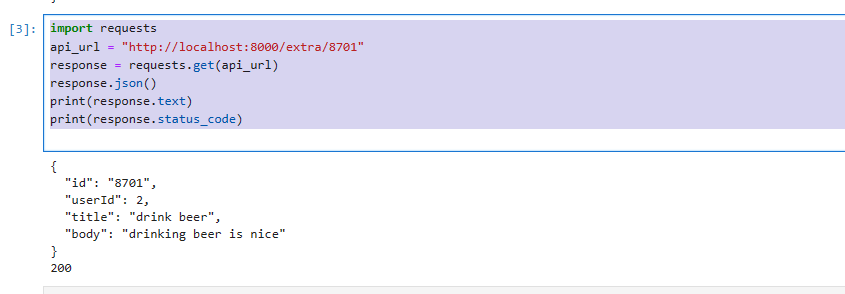
import requests import json url = 'http://localhost:8000/extra/8701' headers = {'Content-Type': 'application/json; charset=UTF-8'} response = requests.delete(url, headers=headers) print(response.status_code) # 200 print(response.ok) # True